


Handling the 4000 Character Limit in SQL NVARCHAR and VARCHAR
0
18
0
Scenario
In SQL Server, you might encounter issues when dealing with large dynamic SQL queries that exceed 4000 characters. This can result in syntax errors and truncation. Here, we address common problems and provide solutions for effectively managing these large strings.
Problem Statement
A common issue arises when a dynamic SQL query exceeds 4000 characters. For example:
DECLARE @SQL NVARCHAR(MAX);
SET @SQL = 'SomeMassiveString > 4000 chars...';
EXEC(@SQL);
In some cases, this may work, but for others, it throws an error due to the 4000 character limit, leading to truncated SQL queries and syntax errors. Additionally, trying to print or view these large queries in SQL Server Management Studio (SSMS) can be challenging.
Misconception About NVARCHAR(MAX)
It's a common misconception that NVARCHAR(MAX) is limited to 4000 characters. In reality, NVARCHAR(MAX) can store up to 2GB of data. The confusion often stems from the use of NVARCHAR(n), where n is between 1 and 4000.
Key Considerations
Storage Limits:
VARCHAR(n) truncates at 8000 characters.
NVARCHAR(n) truncates at 4000 characters.
VARCHAR(MAX) and NVARCHAR(MAX) can store up to 2GB.
String Literals:
Strings with the N prefix (e.g., N'String') are treated as NVARCHAR.
Strings without the N prefix are treated as VARCHAR.
Concatenation:
Concatenating VARCHAR(n) and NVARCHAR(n) results in truncation at 4000 characters due to data type precedence.
Solutions
Use NVARCHAR(MAX) Correctly: Ensure all string literals in your dynamic SQL are prefixed with N to prevent truncation:
DECLARE @SQL NVARCHAR(MAX) = ''; SET @SQL = @SQL + N'SomeMassiveStringPart1' + N'SomeMassiveStringPart2';
Avoid Truncation in Concatenation: Use NVARCHAR(MAX) from the start to ensure proper concatenation without truncation:
DECLARE @SQL NVARCHAR(MAX); SET @SQL = N'SomeMassiveStringPart1' + N'SomeMassiveStringPart2';
Complete Example
Here's a complete example that builds a large dynamic SQL query and executes it without truncation:
DECLARE @SQL NVARCHAR(MAX) = N''; -- Initialize as NVARCHAR(MAX)
-- Build the dynamic SQL query in parts
SET @SQL = @SQL + N'SELECT * FROM LargeTable WHERE Column1 = ''Value1'' ';
SET @SQL = @SQL + N'AND Column2 = ''Value2'' ';
SET @SQL = @SQL + N'AND Column3 = ''Value3'' ';
SET @SQL = @SQL + N'AND Column4 = ''Value4'' ';
SET @SQL = @SQL + N'AND Column5 = ''Value5'' ';
-- Add more parts as needed
-- ...
-- Execute the dynamic SQL query
EXEC sp_executesql @SQL;
-- View the large SQL string in SSMS
SELECT @SQL AS [processing-instruction(x)] FOR XML PATH;
Conclusion
Understanding and correctly handling NVARCHAR and VARCHAR limits in SQL Server is crucial for managing large dynamic SQL queries effectively.
By following the outlined solutions, you can prevent truncation issues, ensuring your SQL queries execute as expected.
This method ensures your queries are built and executed correctly, maintaining the integrity and performance of your database operations.
Related Posts
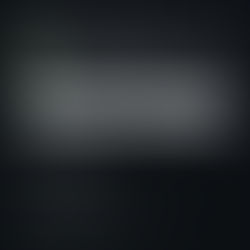

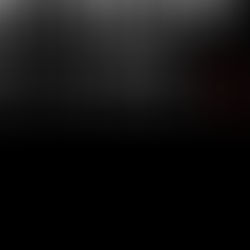












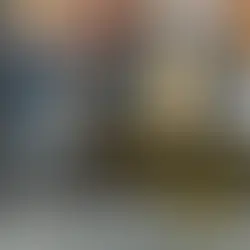


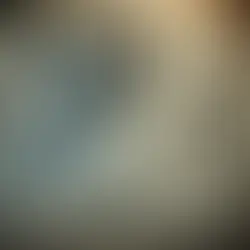



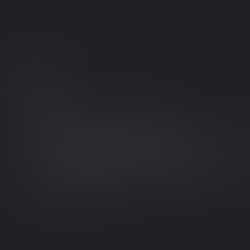





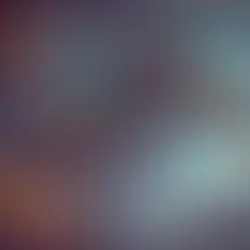

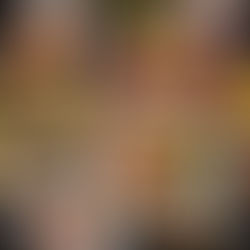


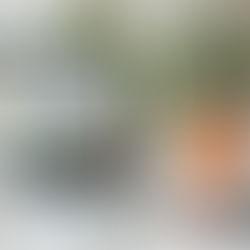

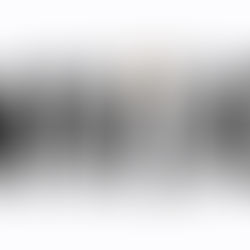

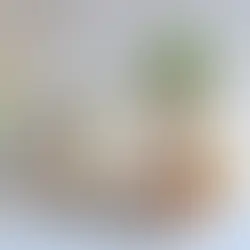

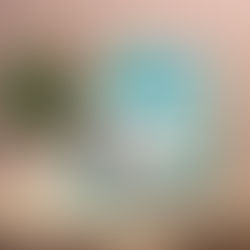

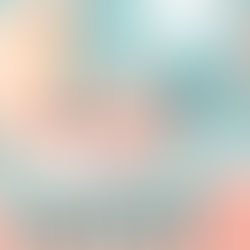



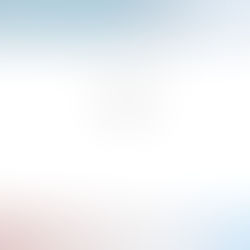


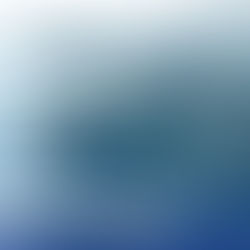

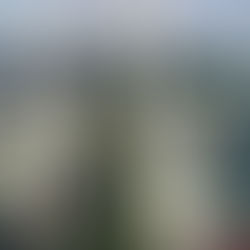

